wsgi-oauth2¶
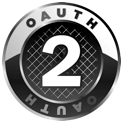
This module provides a simple WSGI middleware that requires the user to authenticate via the specific OAuth 2.0 service e.g. Facebook, Google, GitHub.
Prerequisites¶
It requires Python 2.6, 2.7, 3.2 or higher.
It has no dependencies for non standard libraries, but if there is an installed
simplejson
library, it will be used instead of the standard json
package.
Installation¶
You can install the package via downloading from PyPI:
$ pip install wsgi-oauth2
If you want to use the bleeding edge, install it from the Git repository:
$ pip install git+git://github.com/dahlia/wsgi-oauth2.git
Predefined services¶
There are some predefined services.
-
wsgioauth2.
github
= <wsgioauth2.GitHubService object>¶ (
GitHubService
) The predefined service for GitHub.New in version 0.1.2.
Basic usage¶
from myapp import app
from wsgioauth2 import github
client = github.make_client(client_id='...', client_secret='...')
app = client.wsgi_middleware(app, secret='hmac*secret')
wsgioauth2
— API references¶
-
class
wsgioauth2.
Service
(authorize_endpoint, access_token_endpoint)¶ OAuth 2.0 service provider e.g. Facebook, Google. It takes endpoint urls for authorization and access token gathering APIs.
- Parameters
authorize_endpoint (
basestring
) – api url for authorizationaccess_token_endpoint (
basestring
) – api url for getting access token
-
access_token_endpoint
= None¶ (
basestring
) The API URL for getting access token.
(
basestring
) The API URL for authorization.
-
is_user_allowed
(access_token)¶ Check if the authenticated user is allowed to access the protected application. By default, any authenticated user is allowed access. Override this check to allow the
Service
to further-restrict access based on additional information known by the service.- Parameters
access_token – a valid
AccessToken
New in version 0.1.3.
-
load_username
(access_token)¶ Load a username from the service suitable for the REMOTE_USER variable. A valid
AccessToken
is provided to allow access to authenticated resources provided by the service. If the service supports usernames this method must set the ‘username’ parameter to access_token.- Parameters
access_token – a valid
AccessToken
New in version 0.1.2.
-
make_client
(client_id, client_secret, **extra)¶ Makes a
Client
for the service.- Parameters
client_id (
basestring
,numbers.Integral
) – a client idclient_secret (
basestring
) – client secret key**extra – additional arguments for authorization e.g.
scope='email,read_stream'
- Returns
a client for the service
- Return type
-
class
wsgioauth2.
Client
(service, client_id, client_secret, **extra)¶ Client for
Service
.- Parameters
service – service the client connects to
client_id (
basestring
,numbers.Integral
) – client idclient_secret (:class:basestring`) – client secret key
**extra – additional arguments for authorization e.g.
scope='email,read_stream'
-
client_id
= None¶ (
basestring
) The client id.
-
client_secret
= None¶ (
basestring
) The client secret key.
-
load_username
(access_token)¶ Load a username from the configured service suitable for the REMOTE_USER variable. A valid
AccessToken
is provided to allow access to authenticated resources provided by the service. For GitHub the ‘login’ variable is used.- Parameters
access_token – a valid
AccessToken
New in version 0.1.2.
Makes an authorize URL.
- Parameters
redirect_uri (
basestring
) – callback urlstate (
basestring
) – optional state to get when the user returns to callback
- Returns
generated authorize url
- Return type
basestring
-
request_access_token
(redirect_uri, code)¶ Requests an access token.
- Parameters
redirect_uri (
basestring
) –redirect_uri
that was passed tomake_authorize_url()
code (
code
) – verification code that authorize endpoint provides
- Returns
access token and additional data
- Return type
-
wsgi_middleware
(*args, **kwargs)¶ Wraps a WSGI application.
-
class
wsgioauth2.
AccessToken
(*args, **kwargs)¶ Dictionary that contains access token. It always has
'access_token'
key.-
property
access_token
¶ (
basestring
) Access token.
-
get
(url, headers={})¶ Requests
url
asGET
.- Parameters
headers (
collections.Mapping
) – additional headers
-
post
(url, form={}, headers={})¶ Requests
url
asPOST
.- Parameters
form (
collections.Mapping
) – form dataheaders (
collections.Mapping
) – additional headers
-
property
-
class
wsgioauth2.
WSGIMiddleware
(client, application, secret, path=None, cookie='wsgioauth2sess', set_remote_user=False, forbidden_path=None, forbidden_passthrough=False, login_path=None)¶ WSGI middleware application.
- Parameters
client (
Client
) – oauth2 clientapplication (callable object) – wsgi application
secret (
bytes
) – secret key for generating HMAC signaturepath (
basestring
) – path prefix used for callback. by default, a randomly generated complex path is usedcookie (
basestring
) – cookie name to be used for maintaining the user session. default isDEFAULT_COOKIE
set_remote_user (
bool
) – Set to True to set the REMOTE_USER environment variable to the authenticated username (if supported by theService
)forbidden_path (
basestring
) – What path should be used to display the 403 Forbidden page. Any forbidden user will be redirected to this path and a default 403 Forbidden page will be shown. To override the default Forbidden page see theforbidden_passthrough
option.forbidden_passthrough (
bool
) – Should the forbidden page be passed-through to the protected application. By default, a generic Forbidden page will be generated. Set this toTrue
to pass the request through to the protected application.login_path (
basestring
) – The base path under which login will be required. Any URL starting with this path will trigger the OAuth2 process. The default is ‘/’, meaning that the entire application is protected. To override the default path see thelogin_path
option.
New in version 0.1.4: The
login_path
option.New in version 0.1.3: The
forbidden_path
andforbidden_passthrough
options.New in version 0.1.2: The
set_remote_user
option.-
application
= None¶ (callable object) The wrapped WSGI application.
(
basestring
) The cookie name to be used for maintaining the user session.
-
forbidden
(start_response)¶ Respond with an HTTP 403 Forbidden status.
-
forbidden_passthrough
= None¶ (
bool
) Whether the forbidden page should be passed-through to the protected application. By default, a generic Forbidden page will be generated. Set this toTrue
to pass the request through to the protected application.
-
forbidden_path
= None¶ (
basestring
) The path that is used to display the 403 Forbidden page. Any forbidden user will be redirected to this path and a default 403 Forbidden page will be shown. To override the default Forbidden page see theforbidden_passthrough
option.
-
login_path
= None¶ (
basestring
) The base path under which login will be required. Any URL starting with this path will trigger the OAuth2 process. The default is ‘/’, meaning that the entire application is protected. To override the default path see thelogin_path
option.New in version 0.1.4.
-
path
= None¶ (
basestring
) The path prefix for callback URL. It always starts and ends with'/'
.
-
sign
(value)¶ Generate signature of the given
value
.New in version 0.2.0.
-
class
wsgioauth2.
GitHubService
(allowed_orgs=None)¶ OAuth 2.0 service provider for GitHub with support for getting the authorized username.
- Parameters
allowed_orgs (
basestring
,collections.Container
ofbasestring
) – What GitHub Organizations are allowed to access the protected application.
New in version 0.1.3: The
allowed_orgs
option.New in version 0.1.2.
-
is_user_allowed
(access_token)¶ Check if the authenticated user is allowed to access the protected application. If this
GitHubService
was created with a list of allowed_orgs, the user must be a memeber of one or more of the allowed_orgs to get access. If no allowed_orgs were specified, all authenticated users will be allowed.- Parameters
access_token – a valid
AccessToken
New in version 0.1.3.
-
load_username
(access_token)¶ Load a username from the service suitable for the REMOTE_USER variable. A valid
AccessToken
is provided to allow access to authenticated resources provided by the service. For GitHub the ‘login’ variable is used.- Parameters
access_token – a valid
AccessToken
New in version 0.1.2.
Source code¶
The source code is available under MIT license. Check out from the GitHub:
$ git clone git://github.com/dahlia/wsgi-oauth2.git
We welcome pull requests as well!
Bugs¶
If you found bugs or want to propose some improvement ideas, use the issue tracker.
Author¶
The package is written by Hong Minhee.
Changelog¶
Version 0.2.1¶
Released on September 3, 2020.
Fixed a bug that
ImportError
has raised on Python 3.8. [pull request #12 by Jörg Benesch]Fixed a bug that
GitHubService
raisesKeyError
when users who didn’t declare their name on GitHub tries to login. [pull request #9 by Aymeric Augustin]
Version 0.1.4¶
Released on August 8, 2014.
login_path
can now be configured to protect only a subsection of an application. [pull request #8 by Aymeric Augustin]
Version 0.1.3¶
Released on June 19, 2013.
forbidden_path
can now be configured. Default forbidden page is ugly so also allow forbidden path to be passed through to the protected application so it can be styled properly. [pull request #4 by Mike Milner]GitHubService
now takes an optional parameterallowed_orgs
to limit access based on GitHub organizations. [pull request #4 by Mike Milner]Fix
Client.request_access_token()
to understand Content-Type withcharset
. [pull request #5 by Jacob Kaplan-Moss]
Version 0.1.2¶
Released on March 22, 2013.
Add predefined GitHub service (
wsgioauth2.github
). [pull request #3 by Mike Milner]Add option to set
REMOTE_USER
. [pull request #3 by Mike Milner]
Version 0.1.1¶
Released on May 2, 2012.
Set cookie with
expires
option if the response containsexpires_in
parameter. [pull request #2 by mete0r]
Version 0.1.0¶
Released on November 4, 2011. First version.